Lightbox Image Viewer
Enhances the functionality of images within a rich text block by turning them into a lightbox image viewer.
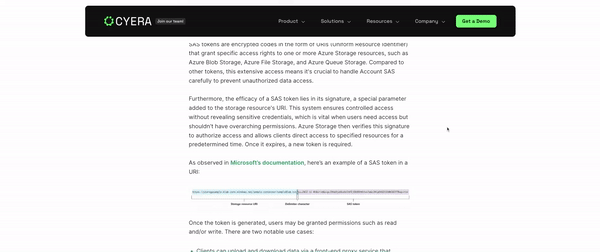
Script
Documentation
This JavaScript code enhances the functionality of images within a rich text block by turning them into a lightbox image viewer. The lightbox viewer allows users to view images in a larger size in a modal dialog overlay without navigating away from the current page. This provides a better user experience for image viewing within a web page.
Usage
To integrate this functionality into your Webflow project:
- Identify Rich Text Block: Add an id attribute with the value #content to the rich text block where you want to enable the lightbox functionality for images.
- Insert JavaScript Code: Paste the provided JavaScript code just before the closing body tag in your Webflow project. This code will automatically detect images within the rich text block with the specified id and enable them for the lightbox viewer.
- Publish and Test: Publish your Webflow project and test the functionality on the live website to ensure that images within the rich text block now act as lightbox images.
Conclusion
By following these simple steps, you can easily enhance the image viewing experience within your Webflow project by enabling images inside rich text blocks to function as lightbox images. This provides users with a convenient way to view images in a larger size without disrupting their browsing experience.